General note
In these series of laboratory exercises you will be learning and practicing various programming concepts enabled by C++ programming language. Mostly, these concepts will be introduced through simple and sometimes complex examples. You are encouraged to think about how and why things work the way they do. The exercises are not set up to trip you.
In order to focus on the task at hand, you can use an integrated development environment (IDE) to write, compile and test your code. In one of the notes provided on Moodle, you can find the steps required to install Code::Blocks on several OS (e.g. Windows, Linux, MacOS). You are encouraged to follow the instructions and install it for your operating system. The operating system on which you develop your code should not matter. All that needs to happen is that your code is C++17-compliant. The system on which your code will be tested has g++
7.5 installed and your code must work on that system (i.e. capa.its.uow.edu.au).
In order to test that your code is compliant and will work on capa.its.uow.edu.au, you will need to do a secure login to capa and test your code. The appropriate option required to ensure that you are compiling against C++17 is the -std=c++17 added to your command line instruction. For example:
g++ file1.cpp file2.cpp file3.cpp -Wall -std=c++17 -o output_exec
If your code does not compile, the maximum mark you can get is 50% of the marks allocated to the exercise. We will not debug your code. Get familiar with your IDE and ensure that the build option is set to use c++17 and turn warning ON with -Wall option.
Use sensible names for your variables and insert comments to let the reader know what your code is doing. If your code contains no comments you will lose marks.
Code submission
For each exercise, name your code as instructed. Each Lab will be due for submission at midnight on Friday of Weeks 3, 5, 7, 9 and 11. You will also be reminded accordingly as the session progresses.
Use the zip archiving tool to pack your codes together and name the zip file your login Lab#.zip; The symbol # represent the lab number. Name your source codes as instructed in each exercise. Place multiple source files required for a give exercise into a folder before archiving all your files. Do not submit project files; only source code files (i.e. .cpp and .hpp).
Task One: Function Template
- Debug file Debug-A.cpp.
- Debug-B.cpp only has one problem, a missing construtor for Add an appropriate one!
- You are given the code findMax.cpp with a function template findMax that uses pass by value Change the code to use pass by reference in this function template. Compile the code and note the error message. What does this error message indicate? In main() function, declare an appropriate data type to call the function template, passing paramters by reference.
Task Two: Another templates
Write code Symbolic.cpp that contains a function template to display a value preceded and succeeded by n elements of a symbol x on a line, with a space on each side of the value. The three arguments to the function template should be: the value, n, and x. Write a main() function that tests the function with char, int, double and string arguments. The output could be, for example,
*** 47 ***
000 39.25 000
aaaa Bob aaaa
Task Three: Container, iterator and function
- Consider the provided file fibT.cpp. What is it trying to do? When will it work on a type? Demonstrate it “working” for at least one type other than unsigned int or
- Look at the source code in One.cpp and in Two.cpp. The idea is for you to think about how some generic functionality fits
- How do the two files differ?
- Compile and run each of the
- What do the programs do?
- Why do the results differ? Is this a good thing? Why or why not?
- Would you typically expect this behaviour when sorting? Why or why not?
Task Four: More template exercises
- Write doubled.cpp to include a function template doubled that can take a type and return the addition of the element to itself, using +.
- Show it working for int, float, char, and Show means you need to demonstrate how the value is in some way doubled.
- What functionality does a type need in order to work with your function doubled and with your demonstration?
- Show it working for one ADT that you
- This activity involves implementing a template class in a file cpp.
- Write a class template hold that holds a single data type of to-be-specified type
- Copy your function template doubled from the previous question into this class
- Demonstrate the use of doubled through an instance of the template class for a range of
- Write a class template Two<R,S> with the two parameterised types R and Write the class template and a main() function with appropriate code to test the following functionality as you add it.
- The class should have a constructor that takes one object of each type and uses those to set the values in the
- There should be a display function that outputs the object
- There should be a function that attempts to add the two variables
What happens if you attempt to instantiate a template class based on this class template for types where at least one of the operations doesn’t make sense?
Task Five: Some generics
- Consider the function template and definitions below.
template <typename T>
T funcExp(T list[], int size){ int j;
T x = list[0];
T y = list[size-1]; for(j=1; j<(size-1)/2;j++){
if (x < list[j]) x = list[j];
if (y > list[size-1-j]) y = list[size-1-j];
}
return (x+y);
}
int list[8]={1,2,9,3,5,8,13,10};
string strlist[]={"one","fish","two","fish","red","fish","blue","fish"};
Determine the output of the following statement, firstly without implementing the code and then check your solution by implementing it in funcExp.cpp.
cout << funcExp(list,8) << " :: " << funcExp(strlist,8) << endl;
Breathe a Sign of Relief with our Academic Assistance: Get instant help, 100% personalized and accurate solutions that make your study life better.
Expert's Answer
Chat with our Experts
Want to contact us directly? No Problem. We are always here for you
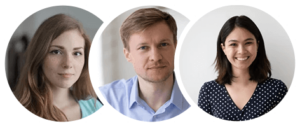
Your future, our responsibilty submit your task on time.
Order NowGet Online
Assignment Help Services