Due: 9:40PM, 10/19
Submission: Upload a text or a Word document that contains your code
For each question, turn in one program only (points earned based on functionality). That is, if you work on 3 questions in total, you should turn in three pieces of code.
Grading: 30 pts (+10 X.C.)
Question 1 [7 pts]
Write a Python program to take an integer input from the user and store the input in variable n. The program prints n multiples of 3 in the descending order, with the last number being 3 [4 pts]. Print the sum and average of the printed numbers [3 pts].
Question 2 [8 pts]
Basic feature [3 pts]
Write a function to perform unit conversion between kilograms (kg) and pounds (lb) (1 kg = 2.205 lb). The function takes 2 arguments—unit (kg or lb) and measurement—and prints the converted value directly inside the function.
Additional Features [1.5/1.5/2 pts]
- The function prints an error message (you design it) when an inappropriate unit (e.g., in) is
- The function is non-case-sensitive to the unit argument (e.g., still runs properly with “KG” or “Kg”).
- Print the original input together with the converted value (see examples below). All Units must be printed in upper
Question 3 [5 pts]
Write a Python program to take a string input from the user. The program manipulates the input and print a string (with exactly 4 characters) per the following rules:
- If the input has 5 characters or more, make a new string using the first two and the last two
- If the input has 4 characters, keep it as
- If the input has 3 characters or less, append (concatenate) an appropriate number of underscore characters (_) to the end such that the new string has exactly 4 characters (including underscore(s)).
Question 4 [10 pts]
Suppose you’re working for NBA as a Python programmer. You are now asked to write a program that converts a player’s height (measured in feet and inches) into the international units (meters and centimeters), based on the following requirements
[Unit conversions] 1 foot = 12 inches; 1 inch = 2.54 cm; 1 m = 100 cm.
- Take a user input. Assume the input must follow the rule below: [3 pts] If your code can handle the regular cases below:
- Kevin Durant’s height is 6 feet and 10 In this case, user will enter 6’10
- LeBron James’ height is 6 feet and 9 inches. In this case, user will enter 6’9
[+3 pts] If your code can also handle the ‘special cases’ below:
- Chris Paul’s height is 6 feet even. In this case, user will simply enter 6’ (instead of 6’0)
- Convert user input into centimeters with 1 decimal place and display
[Hint] 6’10 is equal to 6 feet (= 72 inches) + 10 inches = 82 inches; 1 inch = 2.54 cm. For example, if input is 6’10, your program should display 208.3 cm.
For the special cases, if input is 6’, your program should display 182.9 cm.
- To earn the full credit, your program must meet the additional features below:
- [2 pts] Still works correctly when input is 10 feet or larger (e.g., 50’)
- [2 pts] When the converted measure is >= 1,000 cm, display it in meters with 2 decimal places
(e.g., 50’ => 1524 cm => so you should display 15.24 m).
Bonus Question 1 [+5 pts]
[NBA trivial] Do you know the shortest and the highest players in the NBA history?
The shortest is Muggsy Bogues, listed at 5’3. Three players tied as the tallest, listed at 7’7 each.
Basic Feature [3 pts]
Note: To make the outputs better aligned, insert a tab using escape character (\t).
Write a program to take a user input. Use a while loop to print a height conversion table (foot’inch to cm), starting from 5’3 to 7’7. See below for an example (truncated for brevity; the table should end at 7’7).
Additional Feature [2 pts]
Enhance your code so the program can take one user input (following the same rule above) and then ends the table per that input.
Bonus Question 2 [+5 pts]
Use nested loops to print the exponent table exactly as the one below. No partial credits.
Note: Use escape character (\t) to insert a tab between items.
Expert's Answer
Chat with our Experts
Want to contact us directly? No Problem. We are always here for you
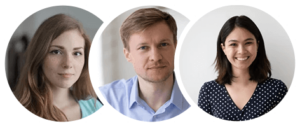
Get Online
Assignment Help Services