Java Code:
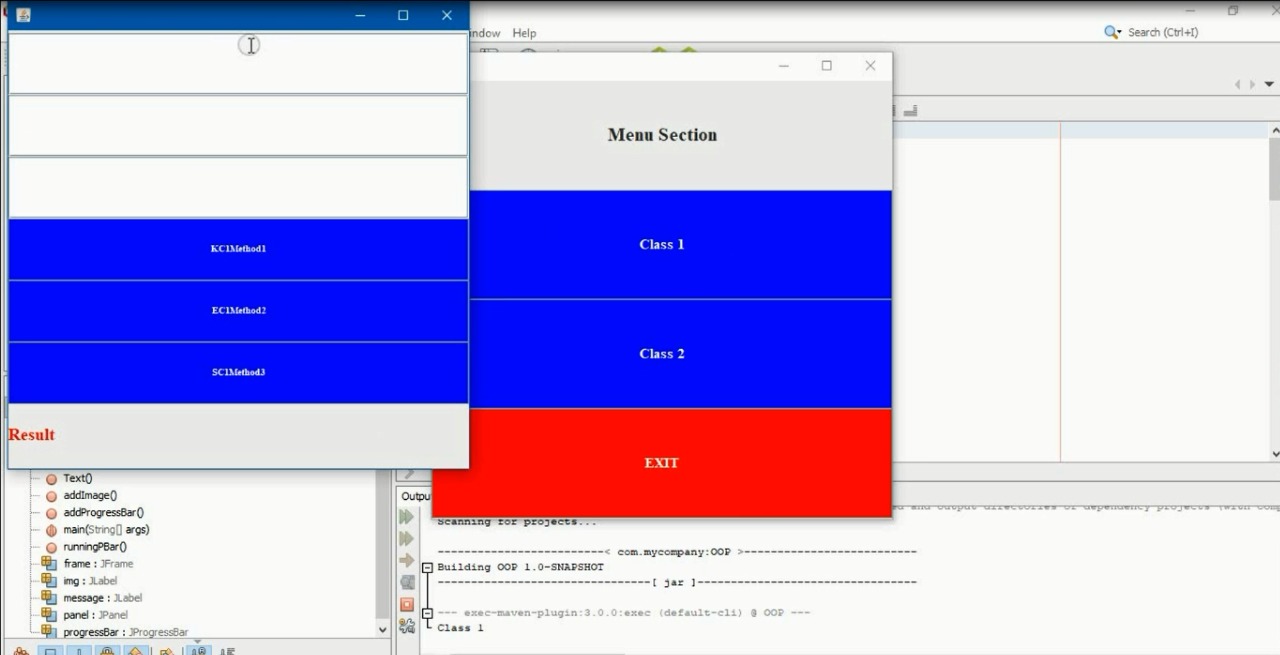
import java.util.ArrayList;
public class AChild2Class extends SParentClass {
private int[] methodClass;
/*
* If The answer is true,Then it will return True String
* else It will return String False
*/
public String TC2Method1(boolean a,boolean b)
{
boolean temp=a & b;
if(temp)
return "True";
else
return "False";
}
/*
* add all values to arrayList
* The return type is ArrayList
*/
private ArrayList<Integer> HC2Method2(int[] a,int[] b,int[] c,int[] d)
{
ArrayList<Integer> temp=new ArrayList<>();
for (int i = 0; i < a.length; i++) {
temp.add(a[i]);
}
for (int i = 0; i < b.length; i++) {
temp.add(b[i]);
}
for (int i = 0; i < c.length; i++) {
temp.add(c[i]);
}
for (int i = 0; i < d.length; i++) {
temp.add(d[i]);
}
return temp;
}
public ArrayList<Integer> AC2Method_2(int[] a,int[] b,int[] c,int[] d)
{
return HC2Method2(a,b,c,d);
}
/*
* This method will add all elements of arraylist
* And find the Sum
*/
public int VC2Method3(ArrayList<Integer> a,ArrayList<Integer> b)
{
int sum=0;
for (Integer integer : a) {
sum=sum+integer;
}
for (Integer integer : b) {
sum=sum+integer;
}
return sum;
}
//constructor
public AChild2Class(int[] child_1_int) {
this.methodClass = child_1_int;
}
//defulat constructor
public AChild2Class() {}
/*
* TASK 5
* return higest value of instance array
*/
public int getChild_1_int() {
int max=methodClass[0];
for (int i : methodClass) {
if(i>max)
max=i;
}
return max;
}
}
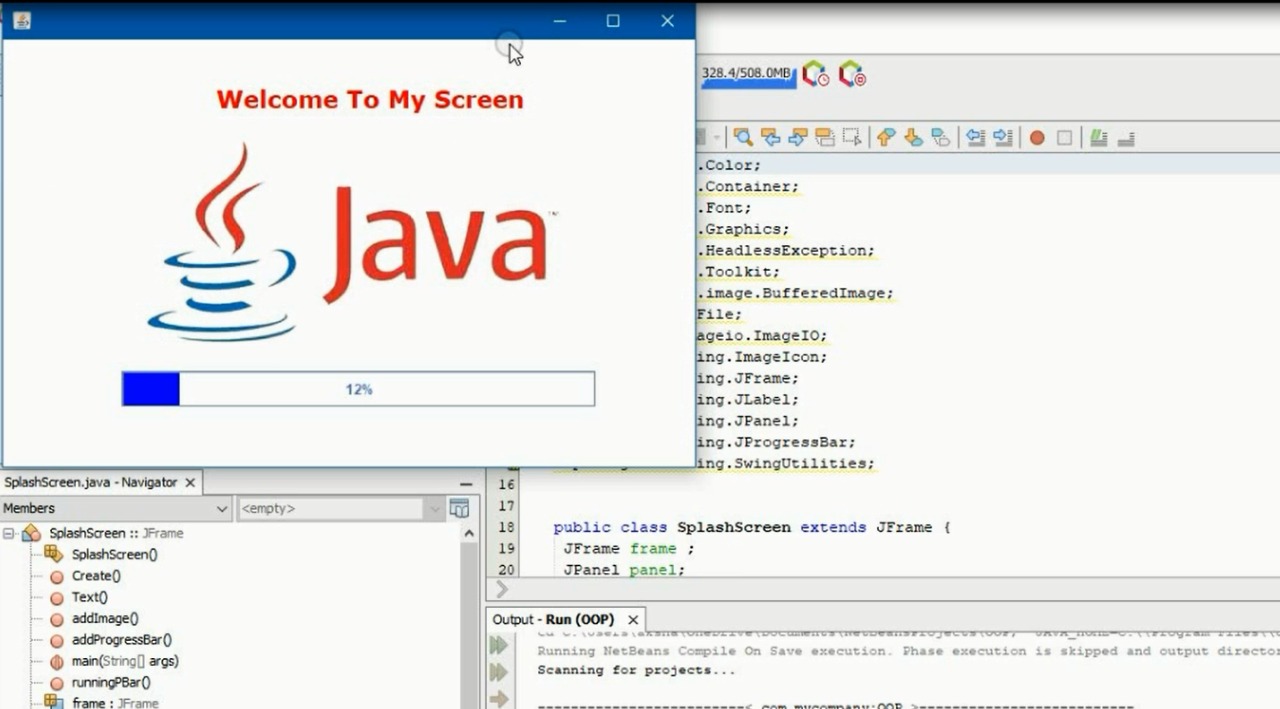
import javax.swing.JFrame;
import javax.swing.JTextField;
import javax.swing.JButton;
import javax.swing.JLabel;
import java.awt.event.ActionListener;
import java.io.FileWriter;
import java.io.IOException;
import java.sql.Timestamp;
import java.time.Instant;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.awt.event.ActionEvent;
import java.awt.Color;
import java.awt.Font;
import java.awt.GridLayout;
Read More
public class class2Child extends JFrame{
JTextField Text1,Text2,Text3,Text4;
JButton C1Method1,C1Method2,C1Method3;
JLabel result;
AChild2Class child2=new AChild2Class();
public class2Child() {
setLayout (new GridLayout(8,1));
Text1 = new JTextField();
Text2 = new JTextField();
Text3 = new JTextField();
Text4 = new JTextField();
C1Method2 = new JButton("TC2Method1");
C1Method2.setBackground(Color.blue);
C1Method2.setForeground(Color.white);
C1Method2.setFont(new Font("Times New Roman", Font.BOLD, 10));
C1Method1 = new JButton("HC2Method2");
C1Method1.setForeground(Color.white);
C1Method1.setBackground(Color.blue);
C1Method1.setFont(new Font("Times New Roman", Font.BOLD, 10));
C1Method3 = new JButton("VC2Method3");
C1Method3.setForeground(Color.white);
C1Method3.setBackground(Color.blue);
C1Method3.setFont(new Font("Times New Roman", Font.BOLD, 10));
result = new JLabel("Result");
result.setFont(new Font("Times New Roman", Font.BOLD, 18));
result.setForeground(Color.RED);
add(Text1);
add(Text2);
add(Text3);
add(Text4);
add(C1Method2);
add(C1Method1);
add(C1Method3);
add(result);
setVisible(true);
C1Method3.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent al) {
String temp=Text1.getText();
String[] res = temp.split("[,]", 0);
int[] a = Arrays.asList(res).stream().mapToInt(Integer::parseInt).toArray();
temp=Text2.getText();
res = temp.split("[,]", 0);
int[] b = Arrays.asList(res).stream().mapToInt(Integer::parseInt).toArray();
ArrayList<Integer>a1=new ArrayList<>();
ArrayList<Integer>b1=new ArrayList<>();
for (int integer : a) {
a1.add(integer);
}
for (int integer : b) {
b1.add(integer);
}
int output=child2.VC2Method3(a1,b1);
result.setText(""+output);
FileWriter myWriter;
try {
myWriter = new FileWriter("TestLog.txt",true);
Timestamp instant= Timestamp.from(Instant.now());
myWriter.write("Time :"+instant.toString());
myWriter.write("nAC2Method3("+Text1.getText()+","+Text2.getText()+")");
myWriter.close();
myWriter.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
});
C1Method1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
boolean aa=true;
boolean bb=true;
int a=Integer.parseInt(Text1.getText());
if(a==0)
aa=false;
int b=Integer.parseInt(Text2.getText());
if(b==0)
bb=false;
String output=child2.TC2Method1(aa,bb);
result.setText(""+output);
FileWriter myWriter;
try {
myWriter = new FileWriter("TestLog.txt",true);
Timestamp instant= Timestamp.from(Instant.now());
myWriter.write("Time :"+instant.toString());
myWriter.write("nTC2Method1("+Text1.getText()+","+Text2.getText()+")");
myWriter.close();
myWriter.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
});
C1Method2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent al) {
String temp=Text1.getText();
String[] res = temp.split("[,]", 0);
int[] a = Arrays.asList(res).stream().mapToInt(Integer::parseInt).toArray();
temp=Text2.getText();
res = temp.split("[,]", 0);
int[] b = Arrays.asList(res).stream().mapToInt(Integer::parseInt).toArray();
temp=Text3.getText();
res = temp.split("[,]", 0);
int[] c = Arrays.asList(res).stream().mapToInt(Integer::parseInt).toArray();
temp=Text4.getText();
res = temp.split("[,]", 0);
int[] d = Arrays.asList(res).stream().mapToInt(Integer::parseInt).toArray();
for (int i : d) {
System.out.println(i);
}
ArrayList<Integer> output=child2.AC2Method_2(a,b,c,d);
String s="";
for (Integer integer : output) {
s+=integer;
s+=",";
}
result.setText(s);
FileWriter myWriter;
try {
myWriter = new FileWriter("TestLog.txt",true);
Timestamp instant= Timestamp.from(Instant.now());
myWriter.write("Time :"+instant.toString());
myWriter.write("nHC2Method2("+Text1.getText()+","+Text2.getText()+","+Text3.getText()+","+Text4.getText()+")");
myWriter.close();
myWriter.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
});
}
}