CSE1OOF Assessment 2 - Create an Object-oriented Design Class Implementation
You will, using object-oriented principles, design a class from a problem description, document that design with a UML diagram, and implement it as a working Java program. Solving it will help you find the answer to life, the universe, and everything! (It’s BQ, of course.)
Specifically, you are tasked with implementing a class that will convert numbers to and from base-26 (Hexaicosadecimal).
The Problem
You’ll all be familiar with our normal base-10 (decimal) number system:
0, 1, 2, 3, 4, 5, 6, 7, 8, 9
You should also be familiar with base-2 (binary), in which there are only two values: 0, 1
There are several other number systems that are important to computer scientists, including base-8 (octal):
0, 1, 2, 3, 4, 5, 6, 7
And base-16 (hexadecimal):
0, 1, 2, 3, 4, 5, 6, 7, 8, 9, a, b, c, d, e, f
I’ve got a typewriter on which, unfortunately, all the number keys are broken. But I still want to do maths! Luckily, all the letter keys work, so I’ve got a handy set of 26 symbols laying around. So I’m in luck: All I have to do is write out my numbers in base-26 (hexaicosadecimal)!
a |
0 |
h |
7 |
o |
14 |
v |
21 |
b |
1 |
i |
8 |
p |
15 |
w |
22 |
c |
2 |
j |
9 |
q |
16 |
x |
23 |
d |
3 |
k |
10 |
r |
17 |
y |
24 |
e |
4 |
l |
11 |
s |
18 |
z |
25 |
f |
5 |
m |
12 |
t |
19 |
||
g |
6 |
n |
13 |
u |
20 |
Now, I’ve gotten pretty good at thinking in hexaicosadecimal, but I keep getting bad grades in maths because nobody else can understand it! Your job is to help me out by writing a Java program that can convert numbers from base-10 to base-26 and vice versa.
Part I: Create your “test harness” (10 marks)
We’ll get to maths in a minute. First, you should create the main() function that you can use to test your program. Create a new class called HexiacosadecimalNumber. Within that class, create your main() function. The main function should behave as follows:
First, it should ask the user to indicate what mode it should be in. If the user types ‘h’ or ‘H’, the program should ask them to input a hexaicosadecimal number (that is, a String). The program should then read that number into a variable, and print it back to the command line (for now). If the user types ‘d’ or ‘D’, the program should ask them to input a decimal number (that is, a double). The program should then read that number into a variable, and print it back to the command line (for now). If the user types ‘q’ or ‘Q’, the program should exit. If the user types anything else, the program should display the message “INVALID INPUT”, and then prompt the user again. The program should then ask the user again what mode it should be in, and repeat the process until the user types ‘q’ or ‘Q’ at the prompt.
To accomplish this, you will need to use a Scanner object in combination with a loop containing an if-else block.
Part II: Add your attributes and methods (10 marks)
Your HexaicosadecimalNumber class should know how to express a number in both hexaicosadecimal and decimal. To do this, it should have two attributes: stringRepresentation and doubleRepresentation. (What types should these variables be, and what should their access modifiers be?)
Your HexaicosadecimalNumber class should have two constructors, both of which will populate the two attributes appropriately. One will take as input a String (representing a number in hexaicosadecimal), and the other will take as input a double. Both methods have to populate both attributes.
You might now be thinking, “How do I populate both attributes when I only have one representation as input to the constructor?” You will need two helper functions: hexaicosadecimalStringToDouble(String in) and doubleToHexaicosadecimalString(double in). You should call these functions in your constructors as needed.
You should also override the toString() method of HexaicosadecimalNumber. This is a special method that belongs to Java’s Object() class, from which all other classes – including HexaicosadecimalNumber – are derived. The signature of the toString() method is
public String toString()
As you can see from the method signature, this function is public, takes no arguments, and returns a String. This function is called automatically when an object of that class is printed, for example by System.out.println().The string should be in the following format:
stringRepresentation (doubleRepresentation)
That is, if your hexaicosadecimal number object is named temp and contains the value abcde (for which the equivalent decimal representation is 19010.0; check this later!), the result of calling
System.out.println(temp)
should be
abcde (19010.0)
For the two “helper” functions - hexaicosadecimalStringToDouble(String in) and doubleToHexaicosadecimalString(double in) – just have them return “dummy” values for now. (I.e. return 0.0 from the first function, and “NOT IMPLEMENTED” from the second.) At this point, you should have a complete working program (that doesn’t do much). Compile and run your program. If you encounter errors, fix them before continuing.
Part III: Convert from a natural hexaicosadecimal number to a double (15 marks) Your next step is to convert a “natural” (that is, whole and zero or positive) hexaicosadecimal number to its equivalent decimal representation. (Where should this code go? Hint: You shouldn’t create any more methods than you already have.) We’ll start with just natural numbers for now to keep things simple, but eventually we’ll be dealing with hexaicosadecimal numbers that are floating point – like floating.point – and/or negative – like -negative.
The algorithm for doing this might look something like:
accumulator = 0
for each character in the input String from left to right
baseValue = the integer equivalent of the current character
accumulator += baseValue * 26length of the whole String – (index of the current character + 1) return accumulator
This is relatively straightforward using appropriate methods from the String class and the Math class, but computing “the integer equivalent of a character” can be a little bit tricky if you’ve never seen it before.
One thing to note is that characters in Java use the Unicode standard, which in turn contains the ASCII standard (a table of ASCII characters can be found here:
https://www.cs.cmu.edu/~pattis/15-1XX/common/handouts/ascii.html)
Another thing to note is that characters in Java are equivalent to their character code. (That is, char c = ‘a’; is exactly the same as char c = 97.)
A third thing to note is that the characters in ASCII are arranged in order. (That is, a = 97, b = 98, c = 99…z = 122).
A fourth thing to note is that while ‘a’ (97) and ‘A’ (65) have different character codes, you already know a convenient function that can ensure that your hexaicosadecimal string is all lowercase (or all uppercase, if you prefer), regardless of how it was typed in.
If you take all these points together, you should be able to extract a single character from the input string and convert it to its equivalent hexaicosadecimal value (That is, a/A = 0, b/B = 1, c/C = 2, … z/Z = 25) simply. (By “simply” I mean one or two short lines of code; if you find yourself wanting to use more than this, perhaps go back to the drawing board.)
Part IV: Convert from a floating point hexaicosadecimal number to a double (20 marks) The next step is to expand our hexaicosadecimal converter to take not just natural numbers, but floating point (a/k/a rational) numbers. The good news is that you’re already halfway done, with your work from the previous section!
What you need to do is wrap your code from the previous section in an if-else block. IF (the input string does not contain a decimal point), do exactly what you did before. IF (the input string DOES contain a decimal point), do what you did before, except where your code had “the length of the whole string”, you’ll instead need “the length of the part of the string that comes before the decimal point.” That will get you the whole number part of the number. Then you’ll need a second loop that looks just at the part of the string that is to the right of the decimal point, and adds those values into the accumulator. (Hint: Negative exponents correspond to dividing instead of multiplying. That is, 10-1 = 0.1 and 26-1 = 0.03846, 10-2 = 0.01 and 26-1 = 0.001479, and so on.)
Part V: Convert from a NEGATIVE WHOLE NUMBER OR FLOATING POINT hexaicosadecimal number to a double (5 marks)
Extend your solution from Part VI to enable conversion of NEGATIVE (that is, beginning with “-“) hexaicosadecimal numbers to doubles.
Part VI: Convert from a double WITHOUT A FRACTIONAL PART to a hexaicosadecimal string (15 marks)
Much like we did with the other method, we’re going to start by solving this problem for the situation of a double that does not have a fractional part – that is, with nothing to the right of the decimal point – and get that working first. Only then (in the next section) will we proceed to solve the general problem of doubles with a fractional part.
The algorithm for doing this might look something like:
temp = the input double converted to an integer type
stringAccumulator = “”
while (temp > 0)
currValue = the remainder when temp is divided by 26
c = currValue converted to the corresponding ASCII value
put c at the beginning of stringAccumulator
temp = the quotient when temp is divided by 26
return stringAccumulator
(NOTE: doubles can contain much bigger values than integer types like int or even long. For the purposes of Part V, you can assume that the value of your double safely fits within the integer type you are using.)
(NOTE on NOTE: To figure out the maximum value that a variable of a given type can take on, you can use the equivalent class. For example, there is a class Integer that provides convenience methods for working with ints, a class Double for working with doubles, a class Long for working with longs, and so on.
Integer: https://docs.oracle.com/javase/7/docs/api/java/lang/Integer.html Double: https://docs.oracle.com/javase/8/docs/api/java/lang/Double.html Long: https://docs.oracle.com/javase/8/docs/api/java/lang/Long.html
These classes have many useful methods for working with values of the associated types. For example, if you want to know the largest value that a variable of a given type can hold, you can access the CLASS_NAME.MAX_VALUE attribute – for example, Integer.MAX_VALUE)
Part VII: Convert from a double WITH OR WITHOUT A FRACTIONAL PART to a hexaicosadecimal string (20 marks)
Again, we’re building on our solution from Part VI, in which we solved for a double that did not have a fractional part. We’re about to relax that restriction, so now our input doubles can have a fractional part as well as a whole number part.
First, we have to get the fractional part. In Part VI, we got the whole number part by converting the double to an integer type. To get the fractional part, all we have to do is subtract the integer from the double.
If the fractional part is zero, the solution is the same as in Part VI. If the fractional part is NON zero, things get a bit trickier. There are multiple ways to solve this problem, but the easiest one is to essentially use the same method we did in Part V. However, before we can use that same algorithm, we have to convert the fractional part to a corresponding integer type value. (For example, if the fractional part was 0.25, the corresponding integer type value would be 25; if the fractional part was 0.12345, the corresponding integer type value would be 12345. In short, you need to move the decimal point to the right end of the number.) If we could do that, we’d be set; we’d just be able to run the algorithm from Part V once on the whole number part, put a “.” in the middle, and then run it again on the corresponding integer for the fractional part.
I’m leaving the rest to you. There are a number of ways to generate the corresponding integer values for a fractional part. You have all the tools you need already; it can be done in a handful of lines with just a loop and basic Java mathematical operations. (But a more elegant solution might be found if you look into other Java classes, such as the BigDecimal class…)
Part VIII: Convert from a NEGATIVE double WITH OR WITHOUT A FRACTIONAL PART to a hexaicosadecimal string (5 marks)
Extend your solution from Part VII to enable conversion of negative doubles to hexaicosadecimal strings.
Expert's Answer
Chat with our Experts
Want to contact us directly? No Problem. We are always here for you
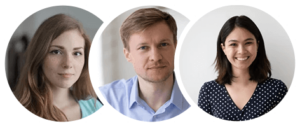
Get Online
Assignment Help Services